As my first attempt at programming a game, I chose to program an 8-bit pong style game. This project helped me to learn much more about procedural programming as well as helping me to understand Visual Basic much better.
Key Aspects
Moving the Paddle
My first job was to program a fully functioning paddle which the user could control. This was relatively straight forward as I was simply able to use the picture boxes built into VB. The next stage was to allow the users to control the paddle. I set up some event handlers which would respond to the “keydown” event. When either the up or down key was pressed, a timer would start. This timer runs every ten milliseconds and moves the paddle up/down by one pixel. The reason I chose to use timers is because it allows the paddle to move much smoother. Having the paddle move every time you press the key down results in staggered movement – unlike the real game. I also added a border, score board and a halfway line to create a nicer design.
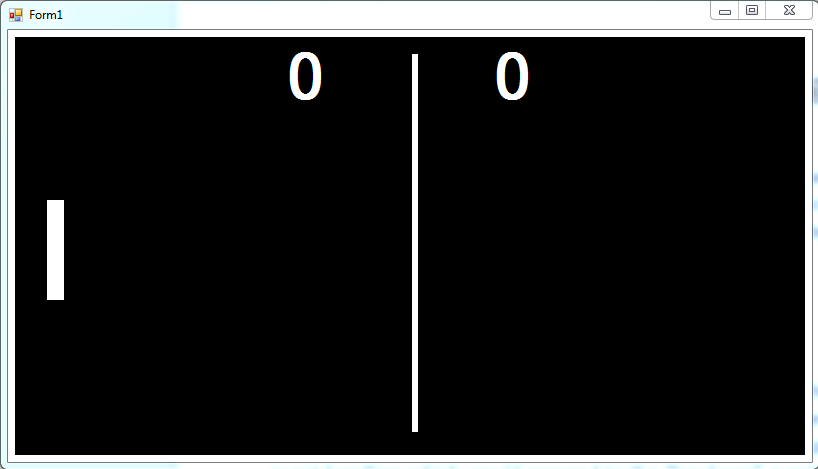
Programming the Ball
The next job was to create a ball for the paddle to hit. Again I simply used a square picture box to display the ball. I also used more timers to move the ball. This time I had ten different timers which each moved the ball in a different direction. The directions mimicked the sixteen point compass directions, for example “EastNorthEast” and “SouthSouthWest.” From the initial kick off, a “West” timer would move the ball left towards the player. After that I split the paddle into five equal sized sections. The section which the ball hit would define what direction the ball would bounce. For example, if the ball hit the very top section of the paddle, it would return with a “NorthNorthEast” direction (most vertically diagonal). This was how I was able to add variation to the movement of the ball. The direction in which the ball bounces off the wall is also dependant on the direction it had when it hit the wall. For example, if the ball hit the wall at a small angle, it would bounce off at a small angle. I now appreciate that the ten different timers is incredibly inefficient and needs improvements however at the time it was great practice for me getting to grips using them.
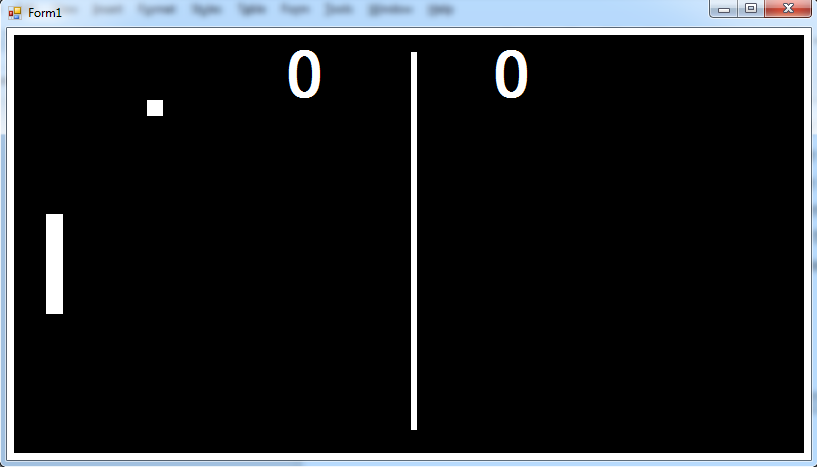
The Enemy
My next task was to program an artificial enemy since I thought making the game two player was too simple. I created the enemy paddle in the same way as the user paddle and set it up similarly so that it is divided into sections which correspond to what direction the ball bounces off it. I then needed some way to automate the movement of the paddle. At this point I realized path prediction was pretty far beyond me and so I created a very simple algorithm which went simply like this:
If ball is above enemy paddle then
Move enemy paddle up
Else if ball is below enemy paddle then
Move enemy paddle down
Else
Keep paddle still
End If
You would be surprised at how much I struggled to beat this algorithm even though I knew exactly what it was going to do.
At this point I had two working paddles and a ball which could get a good rally going. I finally needed to program a scoring system which was very straight forward. If the ball’s X location had passed a certain point, it was beyond a paddle and so the opposition had scored. The score then increments and a kick off is performed which serves to the last conceder. When a player/computer reaches a score of seven it is game over and they win.
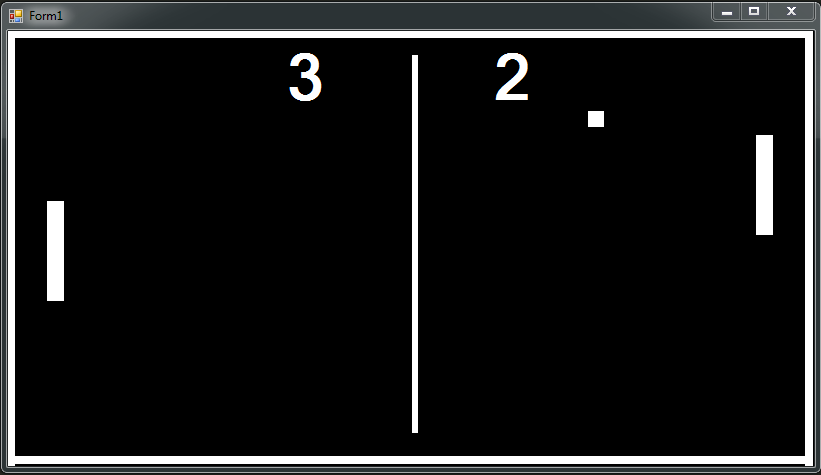
My final job was to create different levels of difficulty for the player. All this entailed was increasing the speed at which the game was played by making the ball and enemy paddle move much quicker. After that, my first game was complete. Nintendo is shaking.
Challenges in Development
Splitting up the Paddle
When the ball hits different sections of the paddle, it should bounce off at different angles so to make the game more realistic. I had trouble doing this. Whilst there is a way to detect picture box intersections in VB, there is no way to return where the intersection has happened. Looking back on this project, my solution was quite terrible however what I decided to do was split the paddle up into five separate picture boxes, each a small square which joined together to make up the paddle. This way I could detect which picture box and part of the paddle has intersected with the ball and therefore which way should the ball bounce. My newly proposed solution would be to stick with the single picture box however also make use of the ‘point’ data type which could track the ball and paddle and determine where the intersection took place when their X values are equal. Unfortunately this is something I did not know much about at the time. This would make the code much more efficient and provide a much smarter solution.
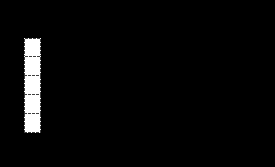
Trouble with Timers
This was a great project for me to learn how to use timers to create real-time applications. Unfortunately that came at the expense of good code. I initially struggled to understand how to use the timers since when one started, it would contradict the other timers. In order to solve this I had to set up a system which would start and stop the appropriate timers instantly when a collision occurred. My approach however was very inefficient. I had too many timers and too much repeated code. Next time around I would have just one timer which controlled the movement of the ball. I would attempt to create a user defined data type which stores the current state/direction of the movement. The movement timer could then use a select case statement to find what state the movement is and change the ball’s X and Y coordinates accordingly.
Areas for Future Improvement
This project was a fantastic way for me to learn lots about VB and bring together everything I had previously learned. Needless to say, however, there are many ways the game could be improved:
-
Much more efficient code – less repetition and better use of subroutines and functions.
-
Better paddle sectioning to remove the need of multiple picture boxes per paddle. The separations in the picture boxes are sometimes visible when the paddle is moving quickly.
-
Making better use of the paint event in VB. This is something I didn’t know much about at the time, however it would have made the program so much more organised. It would remove the need for any picture boxes.
-
Controls created at run time rather than during design. I had all the labels, buttons and picture boxes defined during the design stage of the form. This is incredibly messy and could have been vastly improved by creating the controls during run time.
-
More variation in the movement. Currently there are only ten different directions the ball can travel. It would be much better if the ball used real physics motion equations and angles to give more realistic motion.
-
A better computer enemy. A more sophisticated and less predictable enemy algorithm would make the program much more entertaining to play. Perhaps the algorithm could take into account the current user’s position and aim the ball towards empty spaces.
-
Better difficulty levels. Currently the ball just gets quicker. It would be much more effective if the enemy got smarter and the players could move their paddles quicker.
Conclusion
Regardless of the way it was achieved, I was very happy to have a working game which played somewhat like the real thing. I understood the program and required very little help from the internet to complete it. The reason why I have chosen not to carry out the mentioned improvements is because I have enjoyed benchmarking my progress. It is nice to look back on old programs to help me see my progress. I have no doubt my strengthened knowledge in VB timers will be very useful in future projects.